To increase the brightness of an image, all you need to do is add a value to each component for each pixel in the image.
Increasing the brightness is not always reversible, because as the colour value tops out at 255, if the increased value goes above this value, it will be limited to that value. This makes any reversal of the process impossible. The opposite is true for negative values. Then if the value falls below 0, then the values are limited.
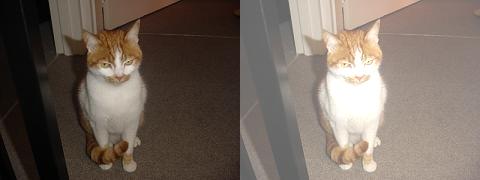
Increasing the brightness by 100
You can download the full code for the sample application which contains code for all the image effects covered in the series here.
public void ApplyBrightness(int brightness) { int A, R, G, B; Color pixelColor; for (int y = 0; y < bitmapImage.Height; y++) { for (int x = 0; x < bitmapImage.Width; x++) { pixelColor = bitmapImage.GetPixel(x, y); A = pixelColor.A; R = pixelColor.R + brightness; if (R > 255) { R = 255; } else if (R < 0) { R = 0; } G = pixelColor.G + brightness; if (G > 255) { G = 255; } else if (G < 0) { G = 0; } B = pixelColor.B + brightness; if (B > 255) { B = 255; } else if (B < 0) { B = 0; } bitmapImage.SetPixel(x, y, Color.FromArgb(A, R, G, B)); } } }
Comments