Contrast in a picture is a measure of how close the range of colours in a picture are. The closer the colours are together, the lower the contrast.
The value for the contrast ranges from -100 to 100, with positive numbers increasing the contrast, and negative numbers decreasing the contrast.
Now, we calculate the contrast value which we will use in the calculation with
((100 + contrast) / 100)2
Then, for each pixel in the image, we take each component of the pixel, and divide the value by 255 to get a value between 0 and 1. We then subtract 0.5, and
any values which are negative will have their contrast decreased, while any positive values will have their contrast increased.
Then we add back the 0.5, and convert the value back to a range of 0-255. After that we set the pixel colour again.
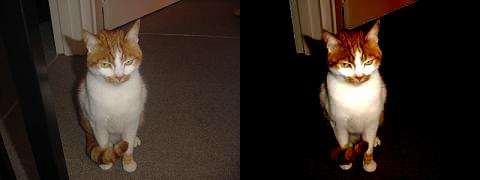
Increasing the contrast by 50
You can download the full code for the sample application which contains code for all the image effects covered in the series here.
public void ApplyContrast(double contrast) { double A, R, G, B; Color pixelColor; contrast = (100.0 + contrast) / 100.0; contrast *= contrast; for (int y = 0; y < bitmapImage.Height; y++) { for (int x = 0; x < bitmapImage.Width; x++) { pixelColor = bitmapImage.GetPixel(x, y); A = pixelColor.A; R = pixelColor.R / 255.0; R -= 0.5; R *= contrast; R += 0.5; R *= 255; if (R > 255) { R = 255; } else if (R < 0) { R = 0; } G = pixelColor.G / 255.0; G -= 0.5; G *= contrast; G += 0.5; G *= 255; if (G > 255) { G = 255; } else if (G < 0) { G = 0; } B = pixelColor.B / 255.0; B -= 0.5; B *= contrast; B += 0.5; B *= 255; if (B > 255) { B = 255; } else if (B < 0) { B = 0; } bitmapImage.SetPixel(x, y, Color.FromArgb((int)A, (int)R, (int)G, (int)B)); } } }
Comments