Gaussian blur also uses convolution to create the effect. If you have not yet read my blog post on Smoothing using convolution, I would advise you to read that post first, as in that post I explain how the convolution function works.
Gaussian blur works very similarly to the smoothing function, in that both are a type of blur, but the Gaussian blur gives a more natural looking blur.
It achieves this by not have a flat weighting, but instead has a weighting based on a Gaussian curve, thus for our 3×3 grid, the values we want to supply the convolution function is as follows:
1 | 2 | 1 |
2 | 4 | 2 |
1 | 2 | 1 |
The factor parameter is set to the sum of each pixel in the grid, which in this case, is again, 16, and the offset is set to 0.
Now we get a nice blur effect.
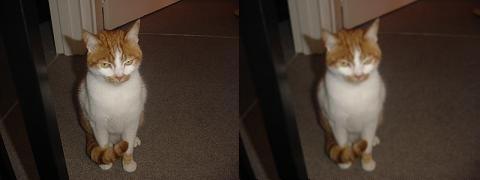
Applying a guassian blur
You can download the full code for the sample application which contains code for all the image effects covered in the series here.
public void ApplyGaussianBlur(double peakValue) { ConvolutionMatrix matrix = new ConvolutionMatrix(3); matrix.SetAll(1); matrix.Matrix[0, 0] = peakValue / 4; matrix.Matrix[1, 0] = peakValue / 2; matrix.Matrix[2, 0] = peakValue / 4; matrix.Matrix[0, 1] = peakValue / 2; matrix.Matrix[1, 1] = peakValue; matrix.Matrix[2, 1] = peakValue / 2; matrix.Matrix[0, 2] = peakValue / 4; matrix.Matrix[1, 2] = peakValue / 2; matrix.Matrix[2, 2] = peakValue / 4; matrix.Factor = peakValue * 4; bitmapImage = Convolution3x3(bitmapImage, matrix); }
Comments