One of the most striking effects to apply to an image is embossing. This is effect is a form of edge-detection, and is also done using convolution, which you can read more about on my post on Smoothing using convolution.
The convolution grid we use in embossing is a little different to the others we have looked at so far. It has an offset of 127, and a fixed factor of 4. The offset makes the the image appear on average as a medium gray.
The grid we will use then is
-1 | 0 | -1 |
0 | 4 | 0 |
-1 | 0 | -1 |
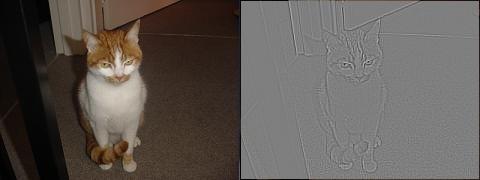
Embossing
You can download the full code for the sample application which contains code for all the image effects covered in the series here.
public void ApplyEmboss(double weight) { ConvolutionMatrix matrix = new ConvolutionMatrix(3); matrix.SetAll(1); matrix.Matrix[0, 0] = -1; matrix.Matrix[1, 0] = 0; matrix.Matrix[2, 0] = -1; matrix.Matrix[0, 1] = 0; matrix.Matrix[1, 1] = weight; matrix.Matrix[2, 1] = 0; matrix.Matrix[0, 2] = -1; matrix.Matrix[1, 2] = 0; matrix.Matrix[2, 2] = -1; matrix.Factor = 4; matrix.Offset = 127; bitmapImage = Convolution3x3(bitmapImage, matrix); }
Comments